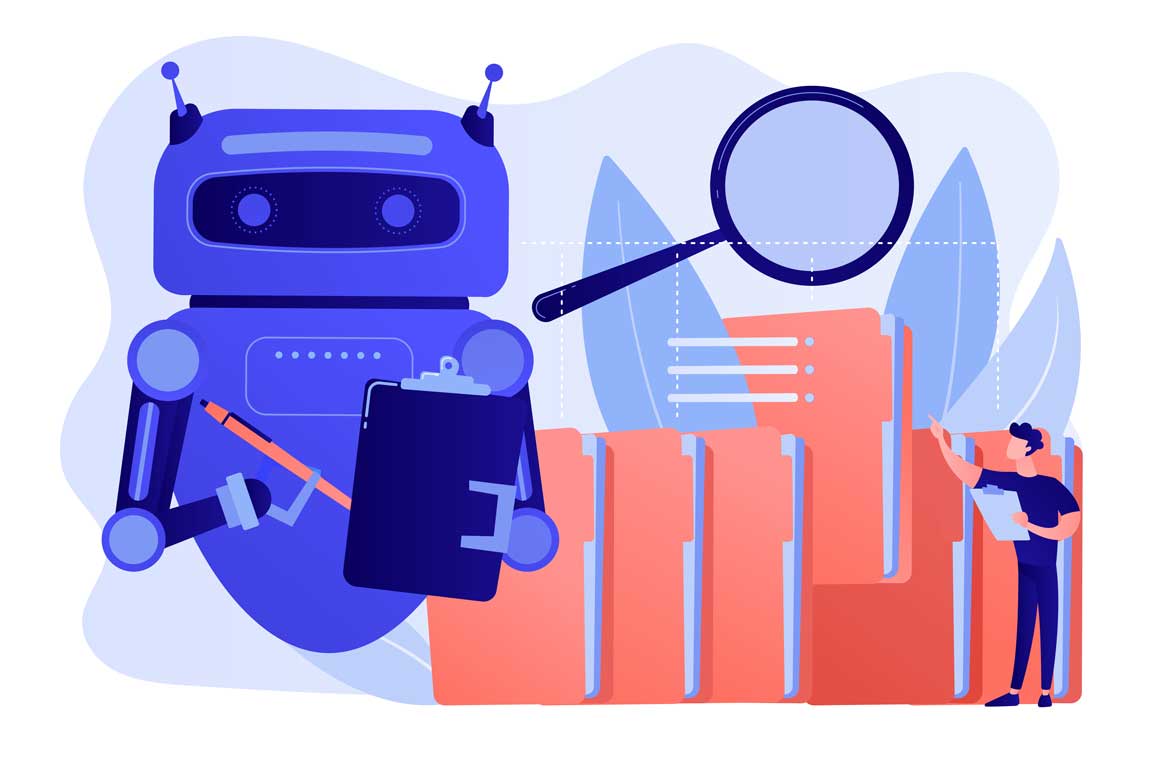
WordPress provides a number of ways to retrieve and display data from its database, and one of the most powerful and flexible methods is the WP_Query class. This class allows developers to create custom loops and display posts and other content based on a wide range of parameters. In this article, we will explore how to use WP_Query and its arguments to create custom loops and display content in your WordPress website.
To use WP_Query, you first need to create an instance of the class and pass it an array of arguments. These arguments allow you to specify the type of content you want to retrieve, the conditions under which it should be retrieved, and how it should be displayed. Some of the most commonly used arguments are:
- post_type: This argument allows you to specify the type of content you want to retrieve. By default, WP_Query will retrieve posts, but you can also use it to retrieve pages, attachments, and custom post types.
- posts_per_page: This argument allows you to specify the number of items you want to retrieve. If you don’t specify a value for this argument, WP_Query will use the default value set in your WordPress settings.
- orderby: This argument allows you to specify how the items should be ordered. You can order the items by title, date, author, etc.
- order: This argument allows you to specify the order in which the items should be displayed. You can display the items in ascending or descending order.
In addition to these arguments, WP_Query also provides a number of other arguments that allow you to fine-tune your custom loop. For example, you can use the meta_query argument to retrieve items based on their custom fields, or the tax_query argument to retrieve items based on their taxonomy terms. A really good resource I use all the time is this website here.
Once you have created an instance of WP_Query and passed it the necessary arguments, you can use it to create a custom loop in your WordPress theme. To do this, you will need to use the have_posts() and the_post() functions. The have_posts() function checks if there are any items that match the criteria specified in the WP_Query arguments, and the the_post() function sets up the current item so that you can access its data.
Here is an example of how you might use WP_Query and its arguments to create a custom loop in your WordPress theme:
$args = array(
'post_type' => 'post',
'posts_per_page' => 10,
'orderby' => 'date',
'order' => 'ASC'
);
$query = new WP_Query( $args );
if ( $query->have_posts() ) {
while ( $query->have_posts() ) {
$query->the_post();
// Display the post content here
}
} else {
// No posts found
}
wp_reset_postdata();
In this example, we are using WP_Query to retrieve the 10 most recent posts and display them in ascending order by date. The wp_reset_postdata() function is used to restore the original post data after the custom loop has finished running.
Why do I have to use wp_reset_postdata()?
The wp_reset_postdata()
function is important because the WP_Query
class modifies the main WordPress query, which can affect the main loop on the page and cause unexpected behavior. By using wp_reset_postdata()
, you can restore the original query and post data, ensuring that the main loop and any other code on the page runs as expected.
Using wp_reset_postdata()
helps to maintain the integrity of the main WordPress query and prevent unexpected side effects from custom loops.